Using APEX Class and Flow
Dynamo task API can be integrated with Salesforce Flow using following APEX code and a supporting VF page.
Visual Force Page
Name: DynamoGetSessionID
<apex:page contentType="text/plain">{!$Api.Session_ID}</apex:page>
APEX class with invocable method
/*
* Helper class to invoke Dynamo Task API from Salesforce Flow.
*/
global class DynamoTaskAPIHelper {
@InvocableMethod(label='Dynamo Task API' description='Dynamo Task API for Flow')
public static List<DynamoAPIResult> callDynamoInvocable(List<DynamoAPIRequest> requestList)
{
DynamoAPIRequest request = requestList[0];
// Requires VF page named "DynamoGetSessionID" with following code:
// <apex:page contentType="text/plain">{!$Api.Session_ID}</apex:page>
// Call VF page to get session ID for API use
String sessionID = Page.DynamoGetSessionID.getContent().toString();
// Optional way of getting session ID, works only for URL triggered flows.
// UserInfo.getSessionId()
String bodyJson = buildBody(sessionID, request.templateID, request.paramId);
String endPoint = request.endPoint;
if (String.isBlank(endPoint))
endPoint = 'https://dynamo-api.documill.com/v1/task';
List<DynamoAPIResult> results = new List<DynamoAPIResult>();
DynamoAPIResult result = new DynamoAPIResult();
sendRequestAsync(bodyJson,UserInfo.getOrganizationId(),request.apiKey,endPoint);
result.status = 'Asynchronous Dynamo API call : ' + bodyJson ;
return results;
}
@future(callout=true)
public static void sendRequestAsync(String bodyJson,String orgId,String apiKey,String endPoint)
{
HttpRequest req = new HttpRequest();
req.setEndpoint(endPoint);
req.setMethod('POST');
req.setTimeout(120000);
req.setHeader('x-dynamo-tenant-id', orgId);
req.setHeader('x-dynamo-api-key', apiKey);
req.setHeader('Content-Type', 'application/json');
req.setBody(bodyJson);
Http http = new Http();
HTTPResponse res = http.send(req);
}
private static String buildBody(String sessionID, String templateID, String paramId)
{
String partnerServerURL = URL.getOrgDomainUrl().toExternalForm() + '/services/Soap/u/50.0/' + UserInfo.getOrganizationId();
List<String> l = new List<String>();
l.add('{');
l.add('"template" : "' + templateID + '",');
l.add('"params" : {');
l.add('"id" : "' + paramId + '"');
l.add('},');
l.add('"integration" : {');
l.add('"type" : "Salesforce",');
l.add('"sessionID" : "' + sessionID + '",');
l.add('"serverURL" : "' + partnerServerURL + '"');
l.add('}');
l.add('}');
String body = String.join(l,'');
return body;
}
global class DynamoAPIRequest {
@InvocableVariable(required=true)
global String templateID;
@InvocableVariable(required=true)
global String paramId;
@InvocableVariable(required=true)
global String apiKey;
@InvocableVariable(required=false)
global String endPoint;
}
global class DynamoAPIResult {
@InvocableVariable
global String status;
}
}
Using With Engage
/*
* Helper class to invoke Dynamo Task API from Salesforce Flow.
*/
global class DynamoTaskAPIHelperEngage {
@InvocableMethod(label='Dynamo Task API Engage' description='Dynamo Task API for Flow')
public static List<DynamoAPIResult> callDynamoInvocable(List<DynamoAPIRequest> requestList)
{
DynamoAPIRequest request = requestList[0];
// Requires VF page named "DynamoGetSessionID" with following code:
// <apex:page contentType="text/plain">{!$Api.Session_ID}</apex:page>
// Call VF page to get session ID for API use
String sessionID = Page.DynamoGetSessionID.getContent().toString();
// Optional way of getting session ID, works only for URL triggered flows.
// UserInfo.getSessionId()
String bodyJson = buildBody(sessionID, request.templateID, request.paramId, request.apiAction);
String endPoint = request.endPoint;
if (String.isBlank(endPoint))
endPoint = 'https://dynamo-api.documill.com/v1/task';
List<DynamoAPIResult> results = new List<DynamoAPIResult>();
DynamoAPIResult result = new DynamoAPIResult();
sendRequestAsync(bodyJson,UserInfo.getOrganizationId(),request.apiKey,endPoint);
result.status = 'Asynchronous Dynamo API call : ' + bodyJson ;
return results;
}
@future(callout=true)
public static void sendRequestAsync(String bodyJson,String orgId,String apiKey,String endPoint)
{
HttpRequest req = new HttpRequest();
req.setEndpoint(endPoint);
req.setMethod('POST');
req.setTimeout(120000);
req.setHeader('x-dynamo-tenant-id', orgId);
req.setHeader('x-dynamo-api-key', apiKey);
req.setHeader('Content-Type', 'application/json');
req.setBody(bodyJson);
Http http = new Http();
HTTPResponse res = http.send(req);
}
private static String buildBody(String sessionID, String templateID, String paramId, String apiAction)
{
String partnerServerURL = URL.getOrgDomainUrl().toExternalForm() + '/services/Soap/u/50.0/' + UserInfo.getOrganizationId();
List<String> l = new List<String>();
l.add('{');
l.add('"template" : "' + templateID + '",');
l.add('"params" : {');
l.add('"id" : "' + paramId + '",');
l.add('"apiAction" : "' + apiAction + '"');
l.add('},');
l.add('"integration" : {');
l.add('"type" : "Salesforce",');
l.add('"sessionID" : "' + sessionID + '",');
l.add('"serverURL" : "' + partnerServerURL + '"');
l.add('}');
l.add('}');
String body = String.join(l,'');
return body;
}
global class DynamoAPIRequest {
@InvocableVariable(required=true)
global String templateID;
@InvocableVariable(required=true)
global String paramId;
@InvocableVariable(required=true)
global String apiKey;
@InvocableVariable(required=false)
global String endPoint;
@InvocableVariable(required=true)
global String apiAction;
}
global class DynamoAPIResult {
@InvocableVariable
global String status;
}
}
When using eSignatures or Approvals, the apiAction parameter should be set to share.
Example of the Apex Code being used in a Salesforce flow.
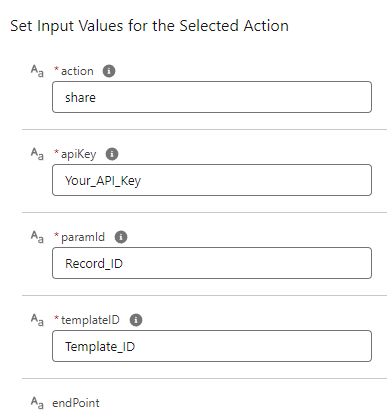
Example Flow
When the previous APEX class and VF page have been added to the org, Salesforce flow can use Apex action “Dynamo Task API” to post tasks.
Add “Action” element and search for “Dynamo Task API”.
Required parameters: template ID, ID parameter value and API key.
Typically ID parameter is used to pass record ID to DAP. In this example flow input variable “recordId” (current record ID) is assigned to ID parameter.
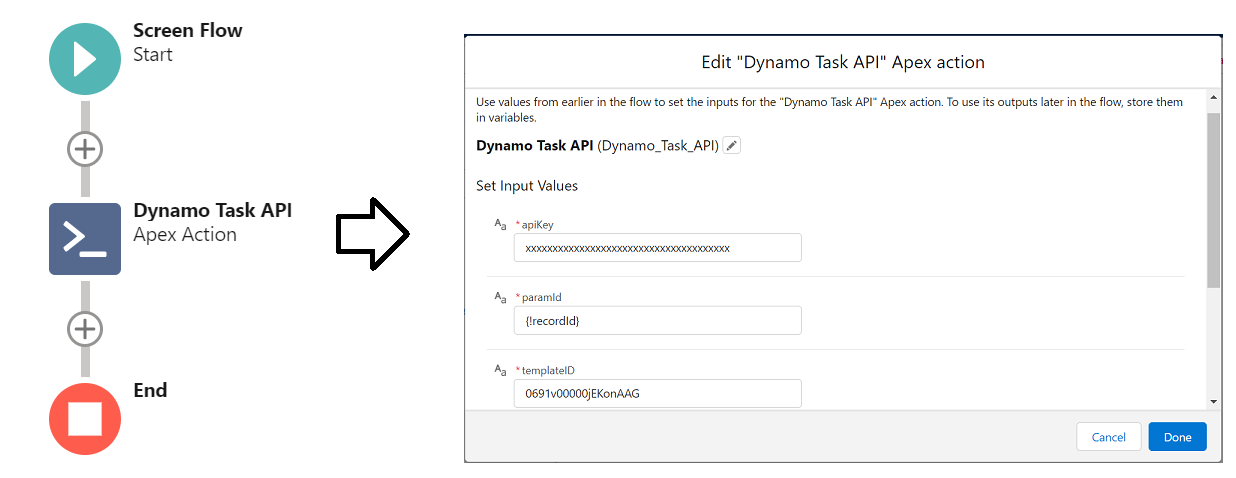